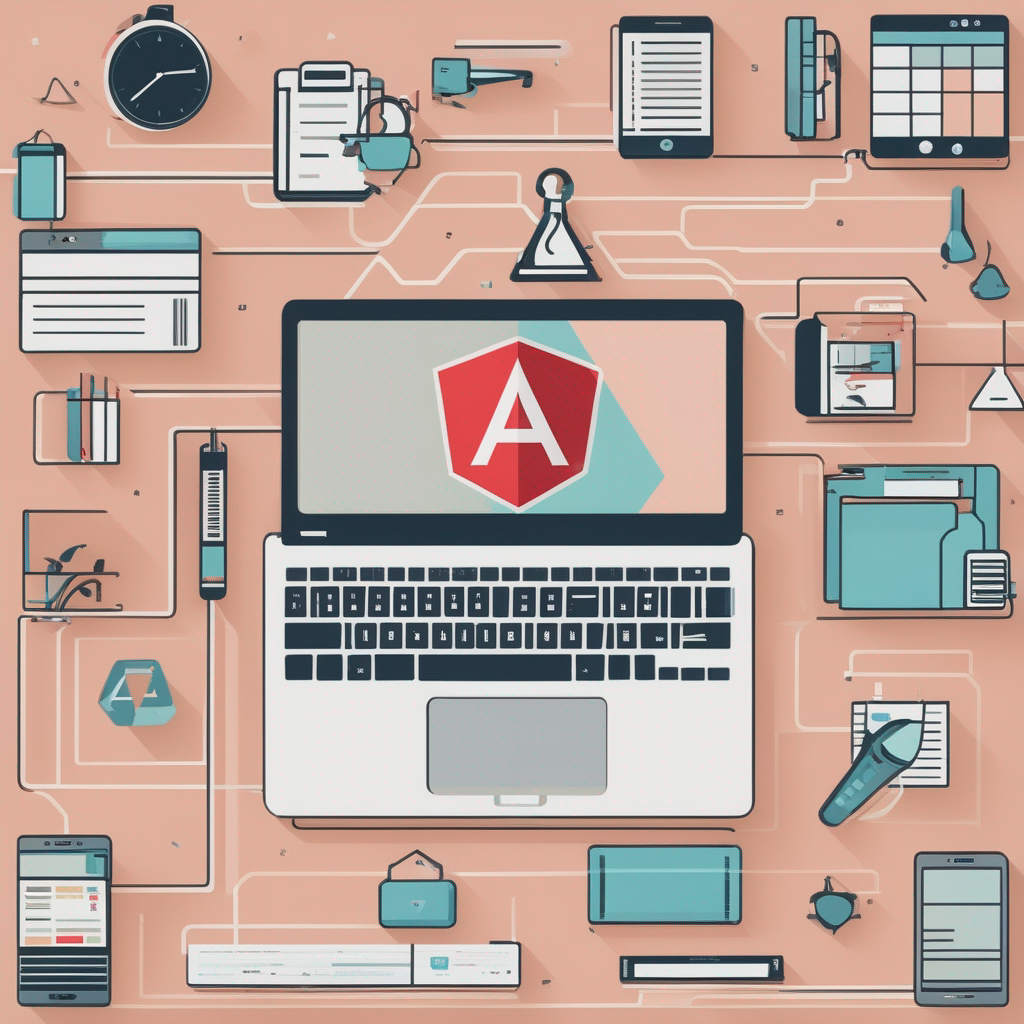
5 Simple Improvements You Can Make To Your AngularJS Code | by Ardy Gallego Dedase | Oct, 2023
[ad_1]
Having built and maintained several Angular apps, there are a few recurring themes I encounter when I rewrite code or review a pull request. I’ll go through five of them and share what we can do about them. I believe most of us are going to encounter similar patterns and potential improvements.
It may seem obvious that we should always unsubscribe from what we subscribe to. However, we can still forget to unsubscribe sometimes. Incomplete observables or unsubscribed subscriptions cause memory leaks. Memory leaks slow down your Angular app.
Instead of calling unsubscribe()
for every subscription, we should handle this automatically to prevent missing unsubscribe()
calls.
What we can do
- If we only need to retrieve the value once, use
first()
ortake(1)
.
const countries$ = ['Canada', 'United States', 'Vietnam', 'Philippines'];
countries$.pipe(take(1)).subscribe(countries => console.log(countries));
2. If you are already using Angular 16, use the takeUntilDestroyed()
operator. It will automatically complete your Observable, ultimately unsubscribing any subscriptions if there are any.
3. If you are using older versions before Angular 16, you can complete or “destroy” your subscription in ngOnDestroy()
. This has the same effect as takeUntilDestroyed()
but with extra boilerplate code.
private destroyed$: ReplaySubject<boolean> = new ReplaySubject(1);ngOnInit() {
this.searchReposFormControl.valueChanges
.pipe(takeUntil(this.destroyed$))
.subscribe((searchString) => {
if (searchString) {
this.searchType = SearchType.REPOS;
this.searchSubject$.next(searchString);
}
});
}
ngOnDestroy() {
this.destroyed$.next(true);
this.destroyed$.unsubscribe();
}
I wrote a blog post that dives deep into this: How to Automatically Unsubscribe Multiple Observables in Angular.
[ad_2]
Source link